What are Mocha and Chai?
Mocha is a JavaScript test framework used by developers around the world. It's an open-source library that anyone can freely use to create their own setup and playback system for automated and manual testing. Mocha runs tests in a sequence, which delivers flexible and accurate reports. Mocha provides functions that execute in a specific order, logging the results in the terminal.
Before starting with the test topic, it is essential to note that this article is only meant for those familiar with Node.js and its underlying technologies.
In this article, you will learn how to create a test suite from scratch using Mocha and Chai, how to run your tests on the command line, and more.
Installing mocha using npm
- Using npm
npm i --global mocha \\ installing globally npm i --save-dev mocha \\ installing as dev dependency
- Using yarn
yarn add mocha
yarn install
Writing tests with Mocha
For writing test cases, we need to have a project or code to test. Let's create a node project using npm.
npm init
Folder Structure π
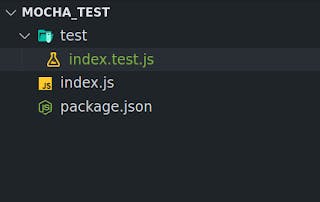
let's put some sample functions to test in index.js
function sum(a, b) {
console.log("adding values ", a, " ", b);
return a + b;
}
function diff(a, b) {
console.log("diff between values ", a, " ", b);
return a - b;
}
function mul(a, b) {
console.log("multiplying values ", a, " ", b);
return a \* b;
}
function div(a, b) {
console.log("dividing values ", a, " ", b);
return a / b;
}
function mod(a, b) {
console.log("modulus values ", a, " ", b);
return a % b;
}
module.exports = {
sum: sum,
diff: diff,
mul: mul,
div: div,
mod: mod
};
Now we can write our first test for our index.js file in the test directory. Mocha tests expect to be placed in the test/.test.js file. Mocha automatically looks for tests inside the test directory of our project.
In the test/ directory, we will create a file named index.test.js to create a test for our index.js.
For assertions, we need another module called chai.
npm i --save-dev chai

writing test π» for the index file function
index.test.js file
const cal = require('../index');
const expect = require('chai').expect;
// creating test suite for one or more tests
describe('testing functions in index.js', function () {
// test a functionality
it('should add numbers : sum function', function () {
let response = cal.sum(1, 2);
expect(response).to.equal(3);
});
it('should diff numbers : diff function', function () {
let response = cal.diff(1, 2);
expect(response).to.equal(-1);
});
});
For running the mocha test. Let's configure package.json for running the mocha test, inside the script field.
"scripts": {
"test": "mocha"
},
Running the test from terminal
npm run test

we run our first test, and everything works fine. but the value of a, and b is hardcoded, we will use the Math.floor(Math.random() * 11) function for generating a and b. For Repeating SetUp we will use the beforeEach hook, Which is used if we have some work we need to do repeatedly for many tests.
beforeEach(function () {
a = Math.floor(Math.random() \* 11);
b = Math.floor(Math.random() \* 11);
});
const cal = require('../index');
const expect = require('chai').expect;
// creating a test suite for one or more tests
describe('testing functions in index.js', function () {
let a;
let b;
beforeEach(function () {
a = Math.floor(Math.random() \* 11);
b = Math.floor(Math.random() \* 11);
});
// test a functionality
it('should add numbers : sum function', function () {
let response = cal.sum(a, b);
expect(response).to.equal(a + b);
});
it('should diff numbers : diff function', function () {
let response = cal.diff(a, b);
expect(response).to.equal(a - b);
});
});
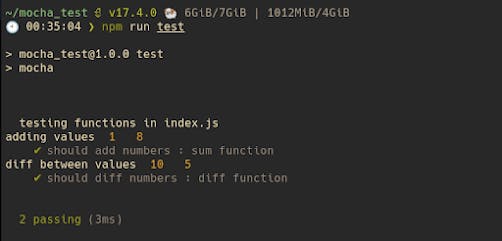